Greetings all, I have spent many days getting a debugger to work using Platformio, OpenOCD, and two Raspberry Picos. Most if not all documentation covers using Visual Code but not PlatformIO integration. I finally figured out you can edit “.code-workspace.json” instead of “launch.json” which gets overwritten. It seems to work and I can step through the initial breakpoints just fine. However, once a task has started, the GDB session stops after the debugger steps through one line. Any help would be greatly appreciated.
Here is my configuration blob:
{
“cwd”: “${workspaceRoot}/openocd”,
“executable”: “${workspaceRoot}/.pio/build/debug/firmware.elf”,
“name”: “Debug with OpenOCD”,
“request”: “launch”,
“type”: “cortex-debug”,
“servertype”: “openocd”,
“gdbPath” : “arm-none-eabi-gdb”,
“device”: “RP2040”,
“configFiles”: [
“interface/picoprobe.cfg”,
“target/rp2040.cfg”
],
sdk/src/src/rp2040/hardware_regs/rp2040.svd",
“svdFile” : “.platformio/packages/framework-arduinopico/pico-sdk/src/rp2040/hardware_regs/rp2040.svd”,
“runToEntryPoint”: “main”,
“preAttachCommands”: [
// “set mem inaccessible-by-default off”
],
“postRestartCommands”: [
“break main”,
“continue”
]
}
Here is my platformio.inf setup:
[env]
platform = "https://github.com/maxgerhardt/platform-raspberrypi.git"
board = pico
framework = arduino
board_build.core = earlephilhower
board_build.filesystem_size = 1m
board_build.f_cpu = 133000000L
monitor_speed = 115200
lib_deps =
adafruit/Adafruit GFX Library@^1.11.3
janelia-arduino/Vector@^1.2.2
adafruit/Adafruit Unified Sensor@^1.1.6
adafruit/Adafruit ADS1X15@^2.4.0
adafruit/RTClib@^2.1.1
arduino-libraries/SD@^1.2.4
greiman/SdFat@^2.2.0
[env:release]
upload_protocol = picotool
[env:debug]
build_type = debug
upload_protocol = picoprobe
debug_tool = picoprobe
What’s your platformio.ini
?
Does regular upload via the picoprobe work?
Yes. that was the 1st sign of success. There are always 30 to 50 fast retries and then is goes through. GDB starts and when the debugger stops at the 1st breakpoint, I can see all the good stuff like local and global data of the module.
We do have documentation though at Using this core with PlatformIO — Arduino-Pico 3.6.0 documentation.
I know we didn’t enable FreeRTOS debugging support in OpenOCD so that it could see FreeRTOS tasks but in general debugging should work. Let me try with the simplest possible FreeRTOS example.
It seems like ESP32 won the popularity competition because there’s a lot of information of that device but not nearly for the Raspberry Pico. I just received the new one with WiFi so hopefully they get some more traction with the community 
Not able to reproduce your issues. Have got
[env:rpipico]
platform = https://github.com/maxgerhardt/platform-raspberrypi.git
board = rpipico
framework = arduino
upload_protocol = picoprobe
debug_tool = picoprobe
and with code src/main.cpp
#include <Arduino.h>
#include <FreeRTOS.h>
#include <task.h>
void blink(void *param) {
(void) param;
pinMode(LED_BUILTIN, OUTPUT);
while (true) {
digitalWrite(LED_BUILTIN, LOW);
delay(750);
digitalWrite(LED_BUILTIN, HIGH);
delay(250);
}
}
void setup() {
Serial.begin(115200);
xTaskCreate(blink, "BLINK", 128, nullptr, 1, nullptr);
}
void loop() { delay(500); }
I can start debugging just fine with PlatformIO only (no cortex-debug extension needed)
Step-over works and I don’t get thrown out after one line.
That’s good news! Are you using two Picos? or a JTAG interface?
I’m using two Picos, one loaded with the Picoprobe firmware, exactly as I’ve detailed and referenced in the documentation.
Ok, which “Type” did you use on your json configuration?
None. PlatformIO autoconfigures it for me.
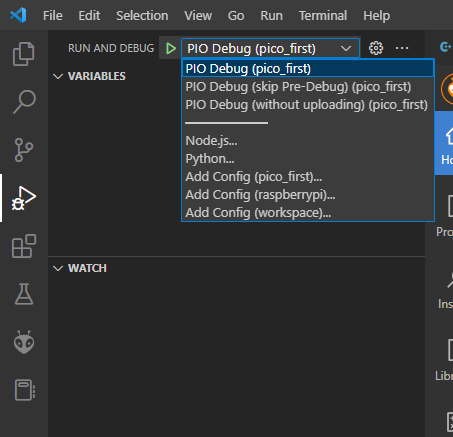
I did not have to manually edit that at all, it all gets derived from the platformio.ini
.
Interesting. I am wondering if I am missing something in the inf settings like build flags in order to use the default type in the launch.json configuration.
Can you reproduce this exact example instead of your project?
Can you reproduce this exact example instead of your project?
Yes, I will do this now. I should have started with that in the 1st place.
Horary! I was able to successfully step through the debugger using the example provided using the default configurations. This tells me there is something bad with my setting and or configurations with the large RTOS project. Thanks for your help, I can now focus on a specific area instead of shotgun hardware, memory, random code and or examples!
1 Like
Great! I’ve looked into also activating FreeRTOS-awareness in OpenOCD, but this seems to not be possible right now without significant OpenOCD modifications, so sadly only seeing the two Cortex-M0+ cores and not each individual FreeRTOS task is the best we can currently do. You’re free to follow the discussion at FreeRTOS + SMP support in OpenOCD · Discussion #1122 · earlephilhower/arduino-pico · GitHub.
1 Like
so sadly only seeing the two Cortex-M0+ cores and not each individual FreeRTOS task is the best we can currently do.
Agreed. It’s like having a V8 but only using 4 cylinders
Greetings Max, I finally figured out what destabilized my debugger! Prior to using a real debugger, I setup a routine at the end of setup() to capture keyboard inputs so I can modify variable while running the device. This routine looked like this:
while(1)
{
while (Serial.available() > 0) {
// Process keyboard inputs
}
yield();
}
Since I now have a real debugger, I no longer need this and it was causing the DGB to throw an exception after stepping through the code via F10 or F5. Thanks again for all your help!
1 Like
Hello,Is it necessary to have two picos for openocd debugging on RP2040? Is it possible to use a daplink and a pico?
If OpenOCD recognizes your debug probe, it’s likely to work. A DAPLink is supposed to be an implementation of the CMSIS-DAP debug adapter protocol, which is exactly what [the firmware}(GitHub - raspberrypi/debugprobe). that you would put on a second Pico implements too. So you should be able to use
upload_protocol = cmsis-dap
debug_tool = cmsis-dap