I finally managed to figure this out. The code below is a routine that builds a char array composed of a loop counter, date & time stamp from the Adafruit Data Logger RTC and x, y & z acceleration values from the Adafruit ADXL345 accelerometer running on Arduino Uno.
Despite the documentation in avr-libc regarding sprintf, I was unable to make char strings to concatenate together - these lines are commented out in the code. Note the “?” in the output below.
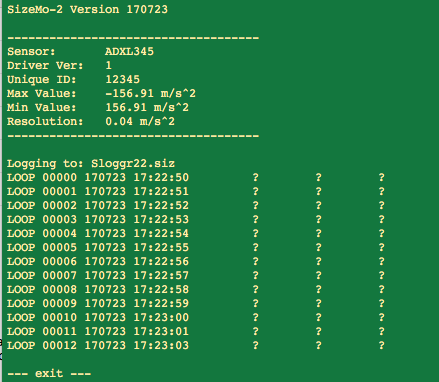
/* Make line out /
char makeLineOut(DateTime now, sensors_event_t e, int nloop)
{
sprintf(kount, “LOOP %05u”, nloop);
strcpy(rcd,kount);
tmp = now.year()-2000;
sprintf(thisYear, " %02u",tmp);
strcat(rcd, thisYear);
sprintf(thisMonth, “%02u”, now.month());
strcat(rcd,thisMonth);
sprintf(thisDay, “%02u”,now.day());
strcat(rcd,thisDay);
tmp8 = now.hour() + 1;
//Add 1 for daylight savings time
sprintf(thisHour, " %02u:“, tmp8);
strcat(rcd, thisHour);
sprintf(thisMinute, “%02u:”, now.minute());
strcat(rcd, thisMinute);
sprintf(thisSecond, “%02u “, now.second());
strcat(rcd, thisSecond);
//Get the sensor readings
// sprintf(x_Val, " %08.3f”, (double)e.acceleration.x);
floatToString(x_Val, e.acceleration.x, prec,width);
strcat(rcd, x_Val);
// sprintf(y_Val, " %08.3f”, (double)e.acceleration.y);
floatToString(y_Val, e.acceleration.y, prec,width);
strcat(rcd, y_Val);
// sprintf(z_Val, " %08.3f”, (double)e.acceleration.z);
floatToString(z_Val, e.acceleration.z, prec,width);
strcat(rcd, z_Val);
// #if ECHO_TO_SERIAL
// Serial.println(rcd);
// #endif
return rcd;
}
Taking SSTAUB’s advice, I searched on the Arduino forum and found the function “floatToString” among other solutions offerend by people facing a similar problem. Using that function to get the 3 float valies for x, y & z in the code above, I now get the correct output below:
Oddly, using the sprintf function, the compiler would throw a warning that I was supplying a float to a function that was expecting a double. Not according to the AVR-libc reference. Casting the float values as doubles got rid of the warning, but still did not produce the correct result. The floatToString function works whether the cast to double is present or not.
According to the Adafruit Sensor template, the acceleration values are given as float.