I would like to build and test a “single source” code on multiple platforms. I’m want to use the same code on a ST Nucleo 32 board and on a LGT8F328P board. But I’m running into problems with pin assignments. So I would like to assign my pins during compilation.
Is there a way I can access the board as specified in the .ini-file from my source code. Be it by a variable or by an IFDEF block?
[env:LGT8F328P-SSOP20]
platform = lgt8f
board = LGT8F328P-SSOP20
framework = arduino
[env:nucleo_l432kc]
platform = ststm32
board = nucleo_l432kc
framework = arduino
Yes, every board implicitly has one or more macros that are given in the build process. When e.g. compiling for your board = nucleo_l432kc
environment, you will see with the project task “Advanced → Verbose Build” that it has the compile flags
arm-none-eabi-gcc -o .pio\build\nucleo_l432kc\FrameworkArduino\pins_arduino.c.o -c -std=gnu11 -mfpu=fpv4-sp-d16 -mfloat-abi=hard -Os -mcpu=cortex-m4 -mthumb -ffunction-sections -fdata-sections -Wall -nostdlib --param max-inline-insns-single=500 -DPLATFORMIO=50200 -DSTM32L432xx -DSTM32L4xx -DARDUINO=10808 -DARDUINO_ARCH_STM32 -DARDUINO_NUCLEO_L432KC -DBOARD_NAME="NUCLEO_L432KC" -DHAL_UART_MODULE_ENABLED […]
So you could use any of those for identification in the code.
#ifdef ARDUINO_NUCLEO_L432KC
/* board = nucleo_l432kc was selected .. */
#endif
#ifdef ARDUINO_ARCH_STM32
/* general STM32-type board / arduino core selected */
#endif
//...
same for the LGT8F328P chip
avr-g++ -o .pio\build\LGT8F328P-SSOP20\src\main.cpp.o -c -fno-exceptions -fno-threadsafe-statics -fpermissive -std=gnu++11 -Os -Wall -ffunction-sections -fdata-sections -flto -mmcu=atmega328p -DPLATFORMIO=50200 -DARDUINO_ARCH_AVR
-DARDUINO_AVR_LARDU_328P -DAVR_LARDU_328E -DF_CPU=32000000L -DCLOCK_SOURCE=1 -DARDUINO_ARCH_AVR -DARDUINO_ARCH_LGT -DARDUINO=10812 -Iinclude -Isrc -IC:\Users\Max.platformio\packages\framework-lgt8fx\cores\lgt8f -IC:\Users\Max.platformio\packages\framework-lgt8fx\variants\lgt8fx8ds20 src\main.cpp
So you can use ARDUINO_ARCH_AVR
, ARDUINO_ARCH_LGT
, ARDUINO_AVR_LARDU_328P
etc.
1 Like
Thanks Max. This is exactly the information I needed. Works like charm.
Hello,
I was looking to create a topic but my issue is close to what was written here before.
I need a name for my board to use it as a macro in my main.cpp.
When I do the same action as @maxgerhardt did, with my project environnement set on arduino uno. I get no name for my board. Is there other way to get the name and used it as macro ?
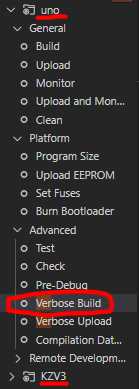
build verbose result
> Executing task in folder ds18b20: C:\Users\xxx\.platformio\penv\Scripts\platformio.exe run --verbose --environment uno <
Processing uno (platform: atmelavr; board: uno; framework: arduino; lib_deps: OneWire@2.3.5, DallasTemperature@3.9.1; src_filter: +<*> -<.git/> -<.svn/> -<test_ds18b20.cpp> +<bus_ds18b20.cpp>) --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------CONFIGURATION: https://docs.platformio.org/page/boards/atmelavr/uno.html
PLATFORM: Atmel AVR (3.3.0) > Arduino Uno
HARDWARE: ATMEGA328P 16MHz, 2KB RAM, 31.50KB Flash
DEBUG: Current (avr-stub) On-board (avr-stub, simavr)
PACKAGES:
- framework-arduino-avr 5.1.0
- toolchain-atmelavr 1.70300.191015 (7.3.0)
LDF: Library Dependency Finder -> http://bit.ly/configure-pio-ldf
LDF Modes: Finder ~ chain, Compatibility ~ soft
Found 18 compatible libraries
Scanning dependencies...
Dependency Graph
|-- <OneWire> 2.3.5 (C:\Users\xxx\Documents\Code\platform\ds18b20\.pio\libdeps\uno\OneWire)
|-- <DallasTemperature> 3.9.1 (C:\Users\xxx\Documents\Code\platform\ds18b20\.pio\libdeps\uno\DallasTemperature)
| |-- <OneWire> 2.3.5 (C:\Users\xxx\Documents\Code\platform\ds18b20\.pio\libdeps\uno\OneWire)
|-- <BoardDefinitionV3_1> (C:\Users\xxx\Documents\Code\platform\ds18b20\lib\BoardDefinitionV3_1)
Building in release mode
MethodWrapper(["checkprogsize"], [".pio\build\uno\firmware.elf"])
Advanced Memory Usage is available via "PlatformIO Home > Project Inspect"
I need to use a macro for two customs boards like this :
ifdef board_custom_1
pinMode(VDD,OUTPUT); //board_custom_1 need this
digitalWrite(VDD,HIGH);
endif
ifdef board_custom_2
pinMode(VDD,OUTPUT); //board_custom_2 need this
digitalWrite(VDD,LOW);
endif
same for including or not some definition.h
ifdef board_custom_1
#include "board_custom_1.h"
endif
ifdef board_custom_2
#include "board_custom_2.h"
endif
Thanks in advance for the help 
You didn’t post your platformio.ini
, without that I can’t tell you what’s wrong.
However, you seem to be going in the wrong direction anyways. If you just have seperate board environments and need different global macros being visible for them, just add build_flags
expression to them.
As e.g.
[env:board1]
platform = atmelavr
; both envs use uno board
board = uno
framework = arduino
build_flags = -D BOARD_CUSTOM_1
[env:board2]
platform = atmelavr
board = uno
framework = arduino
build_flags = -D BOARD_CUSTOM_2
1 Like
That means what exactly, “Getting no name”? The board definition for uno.json
clearly shows
the macro ARDUINO_AVR_UNO
being defined.
Thus you can tell if you selected board = uno
in the platformio.ini
. If multiple environments use the same board
value, differentiate them with the build_flags
options and arbitrary macros above.
That means what exactly, “Getting no name”?
When I go to “Advanced → Verbose Build” I don’t find what macro are available.
Ah, I see. Due to the wrong formatting above the verbose log was cut off early.
The commands are only visible when a source file is built. Here you executed a verbose build even though the firmware was already previously built. Execute a “Clean” and then again a verbose build.
1 Like
Thank you that was exactly my issue 
Sorry I forget to post my platformio.ini file :
Using -DBoard seems to change my build whereas -D seems a more transparent solution regarding the build.
My config below
platformio.ini
; PlatformIO Project Configuration File
;
; Build options: build flags, source filter
; Upload options: custom upload port, speed and extra flags
; Library options: dependencies, extra library storages
; Advanced options: extra scripting
;
; Please visit documentation for the other options and examples
; https://docs.platformio.org/page/projectconf.html
[platformio]
default_envs = KZV3
[common_env_data]
lib_deps =
OneWire@2.3.5
DallasTemperature@3.9.1
src_filter = ${env.src_filter} -<test_ds18b20.cpp> +<bus_ds18b20.cpp>
[env:uno]
platform = atmelavr
board = uno
framework = arduino
lib_deps =${common_env_data.lib_deps}
src_filter =${common_env_data.src_filter}
;build_flags = -DBOARD_NAME= "UNO"
[env:KZV3] ; env :program_via_AVRISP_mkII Kaizenboard V3.1
platform = atmelavr
board = ATmega328P
board_hardware.oscillator = external ; added
board_build.f_cpu = 4000000L ;added 4 MHz
framework = arduino
monitor_port = ${env:ATmega328P.upload_port}
build_flags = -DKZ_V3
;build_flags = -DBOARD_NAME="KZ_V3"
upload_speed = 9600 ;recommended for 4 MHz
upload_port = COM3
upload_protocol= custom
upload_flags =
-C
$PROJECT_PACKAGES_DIR/tool-avrdude/avrdude.conf
-p
$BOARD_MCU
-P
$UPLOAD_PORT
-c
arduino
-b
$UPLOAD_SPEED
; -c stk500v2
upload_command = avrdude $UPLOAD_FLAGS -U flash:w:$SOURCE:i
lib_deps =${common_env_data.lib_deps}
src_filter =${common_env_data.src_filter}
lib_extra_dirs =
/lib